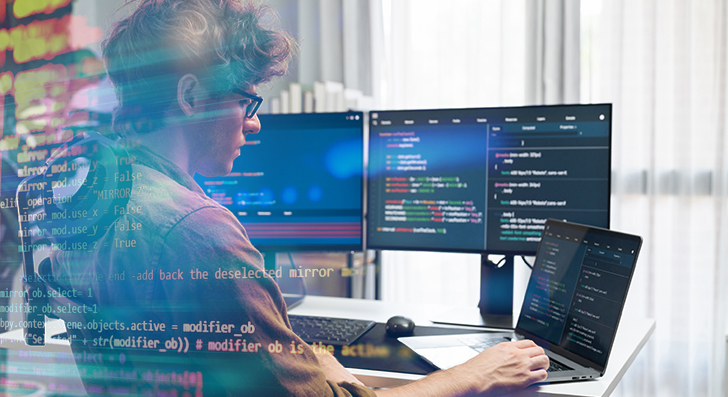
Scalability indicates your software can take care of progress—much more users, a lot more information, and much more traffic—without breaking. For a developer, creating with scalability in mind will save time and tension later. Here’s a transparent and sensible guideline that may help you commence by Gustavo Woltmann.
Design for Scalability from the beginning
Scalability isn't something you bolt on later on—it should be portion of one's plan from the beginning. Many programs are unsuccessful whenever they expand fast due to the fact the original layout can’t handle the extra load. To be a developer, you should Imagine early regarding how your system will behave under pressure.
Start out by developing your architecture being flexible. Keep away from monolithic codebases where by every little thing is tightly related. Alternatively, use modular structure or microservices. These patterns split your application into smaller, independent areas. Each individual module or services can scale By itself with out impacting The full procedure.
Also, take into consideration your databases from working day a person. Will it require to deal with one million customers or perhaps 100? Select the suitable type—relational or NoSQL—according to how your knowledge will improve. Approach for sharding, indexing, and backups early, Even though you don’t need to have them nonetheless.
A further essential stage is in order to avoid hardcoding assumptions. Don’t publish code that only will work underneath present-day situations. Think of what would come about If the consumer foundation doubled tomorrow. Would your application crash? Would the database slow down?
Use style patterns that guidance scaling, like concept queues or celebration-driven techniques. These support your application take care of far more requests devoid of finding overloaded.
Once you Construct with scalability in mind, you're not just making ready for fulfillment—you happen to be minimizing foreseeable future head aches. A nicely-planned method is easier to take care of, adapt, and improve. It’s greater to organize early than to rebuild later.
Use the Right Databases
Selecting the correct database is a vital Component of constructing scalable applications. Not all databases are crafted the exact same, and using the Incorrect you can sluggish you down as well as trigger failures as your application grows.
Start off by comprehension your information. Can it be hugely structured, like rows inside a desk? If Indeed, a relational databases like PostgreSQL or MySQL is a superb in shape. They're strong with associations, transactions, and consistency. Additionally they assistance scaling procedures like go through replicas, indexing, and partitioning to handle far more targeted traffic and information.
If the info is a lot more flexible—like consumer activity logs, merchandise catalogs, or documents—take into account a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are better at dealing with significant volumes of unstructured or semi-structured info and might scale horizontally much more simply.
Also, consider your read through and generate patterns. Will you be undertaking many reads with fewer writes? Use caching and browse replicas. Are you dealing with a significant write load? Explore databases which will handle large write throughput, and even celebration-based information storage programs like Apache Kafka (for momentary details streams).
It’s also intelligent to Feel forward. You might not have to have Innovative scaling capabilities now, but deciding on a databases that supports them means you won’t want to change later on.
Use indexing to hurry up queries. Prevent avoidable joins. Normalize or denormalize your details dependant upon your entry designs. And generally watch databases general performance when you mature.
To put it briefly, the right databases relies on your app’s structure, speed needs, and how you anticipate it to develop. Consider time to pick wisely—it’ll help you save loads of hassle later.
Improve Code and Queries
Speedy code is essential to scalability. As your application grows, each and every tiny delay adds up. Improperly published code or unoptimized queries can decelerate efficiency and overload your process. That’s why it’s essential to Create productive logic from the start.
Get started by crafting cleanse, straightforward code. Steer clear of repeating logic and take away nearly anything unneeded. Don’t choose the most sophisticated solution if a straightforward one particular functions. Keep the features brief, concentrated, and simple to test. Use profiling applications to seek out bottlenecks—spots exactly where your code usually takes far too prolonged to run or works by using a lot of memory.
Next, check out your database queries. These generally slow points down in excess of the code itself. Be certain Each and every question only asks for the information you truly want. Stay clear of Pick *, which fetches everything, and as a substitute choose unique fields. Use indexes to hurry up lookups. And steer clear of executing too many joins, In particular across huge tables.
For those who recognize a similar info staying requested over and over, use caching. Retail outlet the final results quickly making use of instruments like Redis or Memcached so you don’t must repeat high priced functions.
Also, batch your database operations if you can. In lieu of updating a row one by one, update them in groups. This cuts down on overhead and can make your app read more much more productive.
Make sure to exam with big datasets. Code and queries that perform high-quality with a hundred documents might crash once they have to deal with 1 million.
Briefly, scalable apps are quick apps. Keep your code restricted, your queries lean, and use caching when necessary. These methods enable your software continue to be sleek and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your application grows, it's got to handle a lot more consumers and a lot more targeted traffic. If anything goes by just one server, it can immediately turn into a bottleneck. That’s wherever load balancing and caching can be found in. These two equipment aid maintain your app quickly, stable, and scalable.
Load balancing spreads incoming visitors throughout various servers. In place of just one server executing every one of the operate, the load balancer routes consumers to various servers based on availability. This suggests no one server receives overloaded. If one particular server goes down, the load balancer can deliver traffic to the others. Applications like Nginx, HAProxy, or cloud-dependent answers from AWS and Google Cloud make this easy to arrange.
Caching is about storing facts briefly so it can be reused promptly. When consumers request exactly the same information yet again—like a product web site or possibly a profile—you don’t have to fetch it from the databases each time. You could serve it from the cache.
There are 2 typical sorts of caching:
1. Server-side caching (like Redis or Memcached) outlets info in memory for speedy accessibility.
two. Consumer-facet caching (like browser caching or CDN caching) retailers static data files close to the person.
Caching minimizes databases load, improves pace, and will make your application a lot more economical.
Use caching for things that don’t transform frequently. And generally make certain your cache is up-to-date when data does adjust.
To put it briefly, load balancing and caching are straightforward but impressive resources. Jointly, they assist your app tackle a lot more people, stay rapidly, and Get better from issues. If you intend to mature, you'll need equally.
Use Cloud and Container Tools
To construct scalable apps, you would like resources that allow your application grow very easily. That’s the place cloud platforms and containers are available. They offer you flexibility, minimize setup time, and make scaling Substantially smoother.
Cloud platforms like Amazon Website Services (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and companies as you require them. You don’t really have to buy hardware or guess foreseeable future ability. When website traffic improves, you could increase more resources with just a few clicks or automatically utilizing auto-scaling. When visitors drops, you are able to scale down to save money.
These platforms also provide solutions like managed databases, storage, load balancing, and security tools. You'll be able to give attention to creating your app rather than managing infrastructure.
Containers are another vital Resource. A container deals your app and every thing it needs to operate—code, libraries, options—into a single unit. This can make it uncomplicated to maneuver your app among environments, from your notebook to your cloud, devoid of surprises. Docker is the most well-liked tool for this.
Once your application utilizes multiple containers, instruments like Kubernetes allow you to handle them. Kubernetes handles deployment, scaling, and Restoration. If one particular component within your application crashes, it restarts it immediately.
Containers also enable it to be straightforward to individual elements of your application into providers. You can update or scale sections independently, which can be perfect for efficiency and reliability.
In a nutshell, utilizing cloud and container instruments indicates you could scale quickly, deploy easily, and Recuperate immediately when troubles occur. If you prefer your app to improve with out boundaries, start employing these tools early. They preserve time, cut down threat, and assist you stay focused on making, not fixing.
Keep an eye on All the things
In the event you don’t watch your software, you won’t know when items go wrong. Monitoring will help the thing is how your application is carrying out, place difficulties early, and make better decisions as your app grows. It’s a essential Element of building scalable techniques.
Start out by monitoring basic metrics like CPU usage, memory, disk Area, and response time. These inform you how your servers and expert services are accomplishing. Equipment like Prometheus, Grafana, Datadog, or New Relic can assist you accumulate and visualize this data.
Don’t just keep track of your servers—check your app also. Keep watch over just how long it will require for people to load internet pages, how frequently glitches materialize, and where by they manifest. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s happening within your code.
Arrange alerts for vital complications. For example, Should your response time goes above a limit or simply a company goes down, you'll want to get notified straight away. This allows you deal with difficulties rapidly, typically just before customers even discover.
Checking is likewise valuable if you make adjustments. In the event you deploy a new aspect and find out a spike in mistakes or slowdowns, you can roll it back right before it will cause true harm.
As your application grows, website traffic and info increase. Without the need of monitoring, you’ll miss indications of problems until it’s far too late. But with the best tools set up, you remain on top of things.
In brief, checking aids you keep the app reliable and scalable. It’s not almost spotting failures—it’s about comprehension your technique and making sure it really works nicely, even stressed.
Final Feelings
Scalability isn’t just for massive companies. Even modest apps want a solid foundation. By coming up with cautiously, optimizing sensibly, and using the appropriate applications, you'll be able to Establish apps that improve smoothly with no breaking stressed. Begin smaller, think huge, and build sensible.